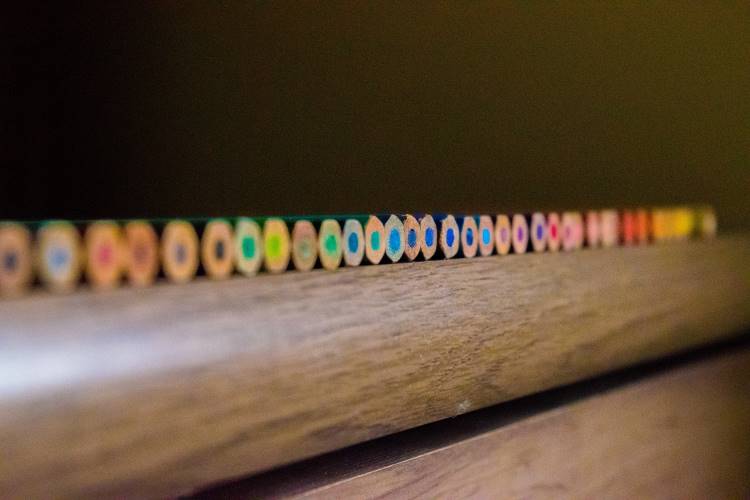
Photo by AVI on Unsplash There is a simple way to add a visual disable state to an HTML element. We just have to add the following CSS class to the parent HTML element we want to disable: .disabled { cursor: initial; pointer-events: none; opacity: 0.5; } Caveats of this method: disabling the elements using […]